Number System Conversion
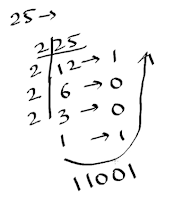
Decimal System: Base 10: Numbers 0 – 9
Binary System: Base 2: Numbers 0 – 1 (Note: Binary numbers are prefixed with “0b”)
>>> bin(25)
'0b11001'
>>> 0b11001
25
>>> bin(410)
'0b110011010'
>>> 0b110011010
410
>>> bin(13)
'0b1101'
>>> 0b1101
13
>>> bin(4)
'0b100'
>>>
>>> 0b100
4
Octal System: Base 8: 0 – 7 (Note: Octal numbers are prefixed with “0o”)
>>> oct(25)
'0o31'
>>> 0o31
25
>>> oct(10)
'0o12'
>>> 0o12
10
>>> oct(256)
'0o400'
>>> 0o400
256
>>> 0o3451
1833
HexaDecimal System: Base 16: 0 – 9 a – f (Note: HexaDecimal numbers are prefixed with “0x”)
Example: Physical Address: 88-78-73-9E-74-38, IPv6 Address: fe80::4c59:48f6:38aa:660%3
>>> hex(8)
'0x8'
>>> hex(9)
'0x9'
>>> hex(10)
'0xa'
>>> hex(16)
'0x10'
>>> hex(25)
'0x19'
>>> hex(100)
'0x64'
>>> 0xf
15
>>> 0x13a
314
>>> 0x243f1
148465
>>> 0x242
578
Configure Python IDLE
In command prompt running Python, we can use the "up key" to see the last command executed. However, in the default setting in Python IDLE the "up key" doesn't show the last command executed.We can configure the IDLE to use the keys as per our choice as shown below:
Python Shell (IDLE) -> Options -> Configure IDLE, and the following dialog box opens to make the settings
Bitwise Operators
>>> ~12-13
>>> bin(12)
'0b1100'
>>> ~1
-2
(~ tilde means Complement Operator)
>>> ~0
-1
>>> 0b00001100
12
>>> 0b11110011
243
>>> bin(-13)
'-0b1101'
>>> 12 & 12
12
(& means Bitwise And)
>>> 12 & 13
12
>>> 12|13
13
(| means Bitwise Or)
>>> 25&30
24
>>> 12&20
4
>>> 3&8
0
>>> 4&8
0
>>> 25|30
31
>>> 12|20
28
>>> 3|8
11
>>> 4|8
12
>>> 12^13
1
(^ means Bitwise XOR)
>>> 25^30
7
>>> 12^20
24
>>> 3^8
11
>>> 4^8
12
>>> 10<<2
40
(<< means Left Shift)
>>> 16<<2
64
>>> 10>>2
2
>>>16>>2
4
(>> means Right Shift)
Import Math Function
The following code to find square root shows error, because Math function is not imported:
>>> x = sqrt(25)
Traceback (most recent call last):
File "<pyshell#0>", line 1, in <module>
x = sqrt(25)
NameError: name 'sqrt' is not defined
In python a number of mathematical operations can be performed with ease by importing a module named “math” which defines various in-built functions which makes our tasks easier.
>>> import math
>>> x = math.sqrt(25)
>>> x
5.0
>>> y = math.sqrt(121)
>>> y
11.0
>>> x = math.sqrt(15)
>>> x
3.872983346207417
>>> print(math.floor(2.4))
2
>>> print(math.floor(2.9))
2
>>> print(math.ceil(2.4))
3
>>> print(math.ceil(2.5))
3
>>> 3**2
9
>>> print(math.pow(3,2))
9.0
>>> print(math.pi)
3.141592653589793
>>> print(math.e)
2.718281828459045
>>> import math as m
>>> m.sqrt(25)
5.0
>>> m.pi
3.141592653589793
>>> m.e
2.718281828459045
>>> m.pow(5,2)
25.0
>>> m.cos(25)
0.9912028118634736
>>> from math import sqrt, pow
>>> sqrt(25)
5.0
>>> pow(3,2)
9.0
>>> pow(4,5)
1024.0
>>> help('math')
Help on built-in module math:
For details on math function, use Python Help command on built-in module math.
Working with PyCharm
Python file extension is .py
To write multiple line of code using IDLE, go to File -> New File
Write the code in the new window. Save it in some location.
To run the code, go to Run -> Run Module:
To run the code through command prompt, use cd to enter the .py file location.
Then type python <file name>.py
Or use the PyCharm IDE
PyCharm is use to run, debug, and trace python scripts and programs.
Or you can use any other IDE like Eclipse, etc.
The benefit of using IDE is, it helps in debugging, by applying breakpoints.
Then select Run -> Debug
To move to next line press F8.
Using Input with PyCharm
x = input("Enter 1st Number: ")
y = input("Enter 2nd Number: ")
z = x + y
print(z)
Result:
Enter 1st Number: 2
Enter 2nd Number: 3
23
The result is 23 because the inputs are taken as strings. We need to convert the inputs to int.
x = input("Enter 1st Number: ")
print(type(x))
Result:
Enter 1st Number: 2
<class 'str'>
x = input("Enter 1st Number: ")
a = int(x)
y = input("Enter 2nd Number: ")
b = int(y)
z = a + b
print(z)
x = int(input("Enter 1st Number: "))
y = int(input("Enter 2nd Number: "))
z = x + y
print(z)
ch = input("Enter a character: ")
print(ch)
Result:
Enter a character: qwe
qwe
But “qwe” is not a character. So, we can use index value to print only the first number.
ch = input("Enter a character: ")
print(ch[0])
Result:
Enter a character: qwe
q
To take the input itself as character only:
ch = input("Enter a character: ")[0]
print(ch)
result = eval(input("Enter an expression: "))
print(result)
Result:
Enter an expression: 2+7-1
8
In the command prompt we can enter inputs using Argument Values argv under system library. The index values are taken as following:
import sys
x = sys.argv[1]
y = sys.argv[2]
z = x + y
print(z)
import sys
x = int(sys.argv[1])
y = int(sys.argv[2])
z = x + y
print(z)The if-elif-else Statement
The following examples shows the use of if-elif-else statements for different purposes.
To find an input number is Odd or Even, with some complement:
x = int(input("Enter a value: "))
r = x % 2
if r == 0:
print("Number is Even")
if x>5:
print("Great")
else:
print("Not so Great")
else:
print("Number is Odd")
print("Bye")
To read an input number:
x = int(input("Enter a value 0-9: ") [0])
if x == 0:
print("Number is Zero")
elif x == 1:
print("Number is One")
elif x == 2:
print("Number is Two")
elif x == 3:
print("Number is Three")
elif x == 4:
print("Number is Four")
elif x == 5:
print("Number is Five")
elif x == 6:
print("Number is Six")
elif x == 7:
print("Number is Seven")
elif x == 8:
print("Number is Eight")
else:
print("Number is Nine")
print("Bye")
To find an integer number is positive or negative:
x = int(input('Enter a non-zero integer: '))
if x == 0:
print('Number is invalid')
elif x > 0:
print('Number is positive')
else:
print('Number is negative')
print('Bye')
To find the largest of three input numbers:
x = int(input("Enter 1st number: "))
y = int(input("Enter 2nd number: "))
z = int(input("Enter 3rd number: "))
if x > y and x > z:
print('Largest number is ', x)
elif y > x and y > z:
print('Largest number is ', y)
else:
print('Largest number is ', z)
print('Bye')
To find the largest of three comma separated input numbers:
nums = input('Enter 3 values x, y, z as comma separated: ')
x, y, z = nums.split(",")
if x > y and x > z:
print('Largest number is ', x)
elif y > x and y > z:
print('Largest number is ', y)
else:
print('Largest number is ', z)
print('Bye')
While Loop
The following examples shows the use of while loop for different purposes.
Printing a text multiple times:
i = 1
while i<=5:
print("Python is awesome!")
i=i+1
Same purpose as above with a different way of iteration:
i = 5
while i>=1:
print("Python is awesome!")
i=i-1
Printing a text multiple times in both dimensions:
i = 1
while i<=5:
print("Python ", end="")
j = 1
while j<=3:
print("Rocks ", end="")
j=j+1
i=i+1
print()
For Loop
The following examples shows the use of for loop for different purposes:
x = 'VINOD'
for i in x:
print(i) # Print all letters of a string
x = ['Vinod', 1202, 7.5]
for i in x: # Print all values of a List
print(i)
for i in 'VINOD': # Putting variable directly within for loop
print(i)
for i in ['Vinod', 1202, 7.5]:
print(i)
for i in range(10):
print(i) # Print all values of a Range from 1 to 10
for i in range(11,30,1):
print(i) # Print all values of a Range from 11 to 30 with 1 increment
for i in range(30,10,-2):
print(i) # Print all values of a Range in reverse order with 2 increments
for i in range(1,50):
if (i%5 != 0): # Do not print number divisible by 5
print(i)
Break – Continue – Pass
The following examples shows the use of break - continue - pass statements for different purposes:
Break
Mimicking a candy machine for user
av = 5
x = int(input('How many candies you want? '))
i=1
while i<=x:
if i>av:
print('Out of stock')
break
print('Candy', i)
i+=1
print('Bye')
Continue
Skip numbers divisible by 3
for i in range(1,101):
if i%3 == 0:
continue
print(i)
print('Bye')
Skip numbers divisible by 3 or 5
for i in range(1,101):
if i%3==0 or i%5==0:
continue
print(i)
print('Bye')
Print only the odd numbers
for i in range(1,101):
if i%2==0:
continue
print(i)
print('Bye')
Print only the even numbers
for i in range(1,101):
if i%2!=0:
continue
print(i)
print('Bye')
Pass
Print only the even numbers
for i in range(1,101):
if i%2!=0:
pass
else:
print(i)
print('Bye')
Print only the odd numbers
for i in range(1,101):
if i%2==0:
pass
else:
print(i)
print('Bye')
To understand the difference between continue and pass, check the outputs of these two codes:
for i in range(1,11,1):
if i%2==0:
pass
print(i)
print('End')
for i in range(1,11,1):
if i%2==0:
continue
print(i)
print('End')
Printing Patterns
The following examples show use of for loop to print patterns:
for i in range(4):
for j in range(4):
print('# ', end="")
print()
for i in range(4):
for j in range(i+1):
print('# ', end="")
print()
for i in range(4):
for j in range(4-i):
print('# ', end="")
print()
n = 4
for i in range(0,n):
for j in range(0,n-i-1):
print(end=" ")
for j in range(0,i+1):
print("* ", end="")
print()
Increase the value of n and see the results.
Some other pattern printing examples are shown below:
for i in range(4):
for j in range(4):
if(i<j):
print(chr(65+14+j), end=" ")
else:
print(chr(65+j), end=" ")
print()
for i in range(4):
for j in range(4):
print(j+i+1, end=" ")
print()
for i in range(4):
for j in range(4-i):
print(j+i+1, end=" ")
print()
n=5
for i in range(0,n):
for j in range(0, n-i-1):
print('*', end=" ")
print()
Reference: Taking online class from Navin Reddy's Python YouTube Videos